Creating Stunning Animated Bubble Charts with JavaScript for Business Insights
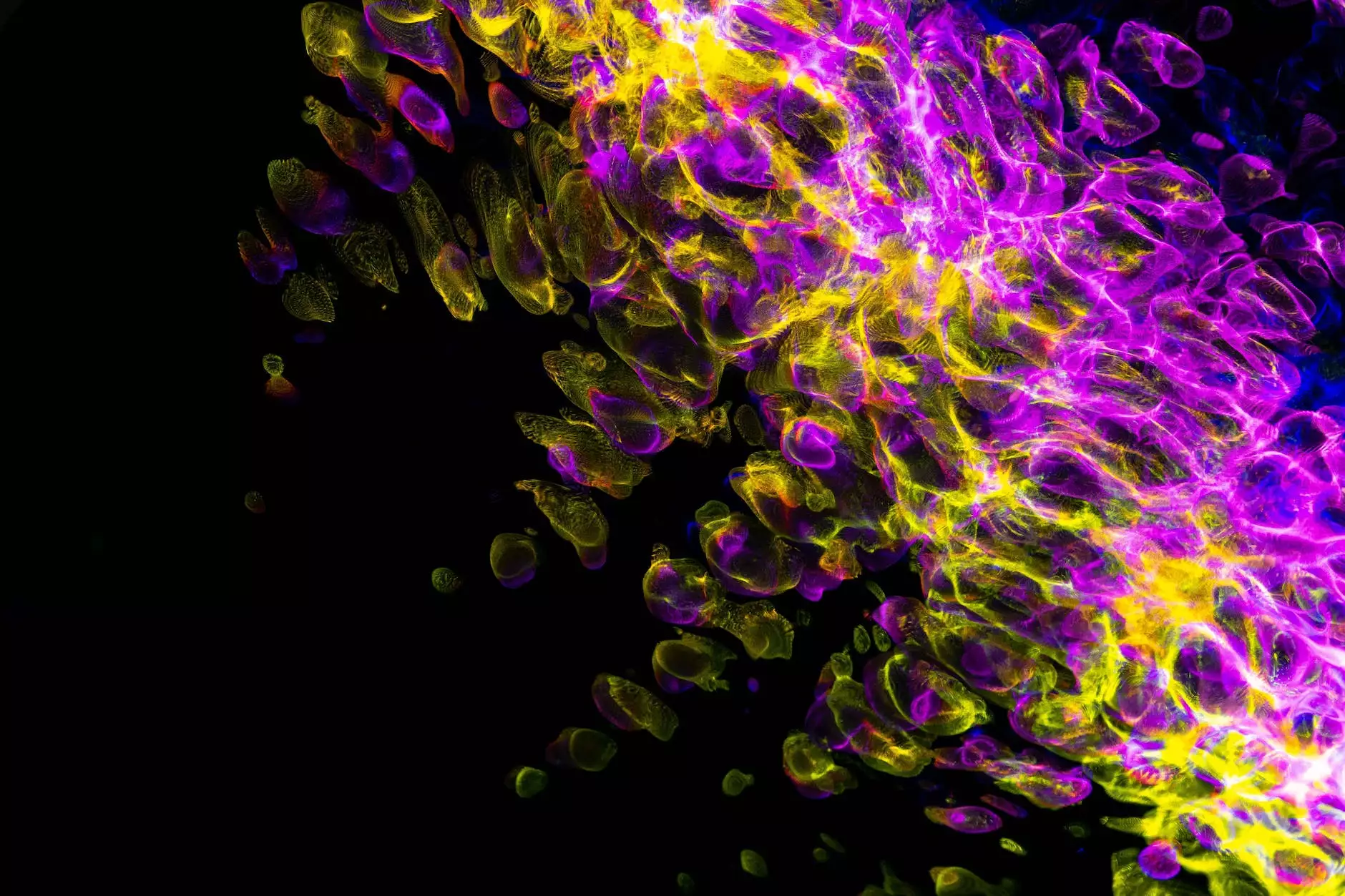
The world of business is becoming increasingly data-driven, and the success of many companies hinges on their ability to analyze and visualize data effectively. One of the most impactful tools in a marketer's arsenal is the animated bubble chart. This powerful visualization combines simplicity and elegance, allowing businesses to showcase complex data in an appealing and comprehensible way. In this article, we will explore how to create stunning animated bubble charts using JavaScript libraries, and how these charts can provide valuable insights into your business strategies.
What is an Animated Bubble Chart?
An animated bubble chart is a type of data visualization that displays three dimensions of data. Each bubble represents a data point, and the position of the bubble relative to the axes indicates two data dimensions, while the size of the bubble indicates the third dimension. The animation enhances the viewer's experience, making it easier to understand trends and changes over time.
The use of animated bubble charts is particularly advantageous in the fields of marketing and business consulting. They can effectively visualize customer segmentation, market research data, and competitive analysis, guiding strategic decisions with clarity and precision.
Benefits of Using Animated Bubble Charts
- Enhanced Data Presentation: Animated bubble charts provide a dynamic way to present complex data sets, making them more engaging to the audience.
- Trend Visualization: Animation allows for the easy identification of trends over time, helping businesses to make data-driven decisions.
- Insightful Data Analysis: By representing three dimensions in one chart, businesses can gain deeper insights that may be overlooked in more traditional visualizations.
- Interactive Experience: Many JavaScript libraries allow users to interact with the bubbles, providing tooltips and additional information on hover, further enriching the user experience.
Popular JavaScript Libraries for Creating Animated Bubble Charts
When it comes to creating animated bubble charts, several JavaScript libraries stand out. Each has its own strengths and use cases. Here are two of the most popular options:
D3.js
D3.js (Data-Driven Documents) is a powerful JavaScript library that uses HTML, SVG, and CSS to bring data to life. With D3.js, you can create highly customizable animated bubble charts that respond to your data inputs.
Features of D3.js:
- Customizable animations for various data states
- Supports a wide range of data formats
- Integration with other visualization libraries
- Robust community support and extensive documentation
Chart.js
Chart.js is another excellent library that is simpler to use than D3.js, particularly for those who are new to data visualization. While it may have fewer customization options compared to D3.js, it serves wonderfully for quick implementations of animated bubble charts.
Features of Chart.js:
- Easy setup with straightforward API
- Responsive designs that suit various devices
- Built-in animations that enhance the presentation of data
- Can easily be integrated with other JavaScript frameworks
How to Create an Animated Bubble Chart Using D3.js
Creating an animated bubble chart with D3.js involves several steps, ranging from setting up your workspace to coding the actual chart. Below, we outline a step-by-step tutorial to guide you through the process.
Step 1: Setting Up Your Environment
Before you can start coding, ensure you have the following:
- A code editor (like Visual Studio Code or Sublime Text)
- A basic understanding of HTML, CSS, and JavaScript
- The D3.js library included in your project, which can be added via a CDN:
Step 2: Prepare Your Data
Your data should be structured in a way that D3 can parse it easily. For this example, we will use a simple dataset with fictional business metrics:
const data = [ {x: 30, y: 30, radius: 20, label: 'Market A'}, {x: 70, y: 70, radius: 30, label: 'Market B'}, {x: 110, y: 50, radius: 25, label: 'Market C'}, ];Step 3: Create the SVG Canvas
In your HTML document, create an SVG element where the chart will be drawn:
Step 4: Draw the Bubbles
Next, use D3.js to bind your data to the SVG and create the bubbles. Here’s how you can do it:
const svg = d3.select("svg"); svg.selectAll("circle") .data(data) .enter() .append("circle") .attr("cx", d => d.x) .attr("cy", d => d.y) .attr("r", d => d.radius) .style("fill", "blue") .style("opacity", 0.7) .on("mouseover", function(event, d) { d3.select(this).style("fill", "orange"); svg.append("text") .attr("x", d.x) .attr("y", d.y) .text(d.label); }) .on("mouseout", function() { d3.select(this).style("fill", "blue"); svg.selectAll("text").remove(); });Step 5: Add Animation
To animate the bubbles, you can introduce transitions. For instance, moving the bubbles upward when they are created:
svg.selectAll("circle") .transition() .duration(2000) .attr("cy", d => d.y - 20) .transition() .duration(2000) .attr("cy", d => d.y);Best Practices for Using Animated Bubble Charts in Business
While animated bubble charts are powerful, there are some best practices to keep in mind when using them in your marketing and consulting reports:
Choose the Right Data
Ensure that the data you are visualizing is meaningful and relevant to your audience. Properly chosen data points can make a significant difference in understanding trends and insights.
Keep It Simple
Avoid cluttering the chart with excessive information. The main goal should be to convey information clearly. Simplifying your visual can often lead to better insights.
Use Clear Labels and Legends
Labels should be easy to read and understand. Provide legends and tooltips where necessary to enhance the interpretability of the data presented.
Test with Your Audience
Before finalizing your chart, consider testing it with a sample audience. Gathering feedback can help refine the visual presentation and ensure it communicates the intended insights effectively.
Conclusion
In today's data-centric business environment, the ability to visualize complex information clearly is paramount. By using tools like JavaScript with libraries such as D3.js and Chart.js, businesses can create animated bubble charts that not only present data but also engage their audience in a meaningful way. As you implement these techniques in your reports and presentations, remember that clarity, simplicity, and relevance are key to making data-driven decisions that can propel your business forward.
Whether you are in marketing or business consulting, understanding how to leverage animated bubble charts can significantly enhance your analytical capabilities. Start experimenting with your data and watch how such visualizations can lead to deeper insights and improved business outcomes.
animated bubble chart javascript